fezder
Well-Known Member
Doggy, I used serial port to debug on where in fontmap is it reading for fontmap: this is from working matrix and indeed it reads like should for character 5:
and output with hex values (as fontmap used also hex so easier to compare)
now then, this new one, it READS from current place from fontmap, but draws 0 on all values between 0-9, but find correct value on fontmap according to serial monitor
? so halfway it works, like reading english but talking japanese lol
and here's reading:
and full code:
C:
void charput(unsigned char ch, signed char x, signed char y)
{
signed char x1, y1;
unsigned char disp;
unsigned char disp2;
for ( x1 = 0; x1 < 8; x1++) // eight rows
{
disp = font[x1 + (ch * 8)];
Serial.println(disp, HEX);
for (y1 = 0; y1 < 8; y1++) // eight pixels
{
disp2 = disp & power[y1];
if (disp2 > 0)
{
pixel(x + x1, y + y1, 0); // OR the pixel to the display buffer
}
}
}
}
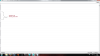
now then, this new one, it READS from current place from fontmap, but draws 0 on all values between 0-9, but find correct value on fontmap according to serial monitor

C:
void charput(unsigned char ch, signed char x, signed char y)
{
signed char y1;
unsigned char disp;
unsigned char disp2;
//for ( x1 = 0; x1 < 8; x1++) // eight rows, still eight bits to load so no changes
//{
// disp = font[x1 + (ch * 8)];
disp = font[ch];
Serial.println(disp,BIN); //display as BINARY format (Bxxxxxxxx) as fontmap is in binary format
for (y1 = 0; y1 < 8; y1++) // eight pixels
{
disp2 = disp & power[y1];
if (disp2 > 0)
{
//pixel(x + x1, y + y1, 0); // OR the pixel to the display buffer
pixel(x, y + y1, 0); // OR the pixel to the display buffer
}
}
//}
}
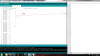
and full code:
C:
#include "FontMap7Segment.h"
int dataPin = 2; // ic: 14, ser_in Define which pins will be used for the Shift Register control
int latchPin = 3; // ic:12 silkscreen numbers!
int clockPin = 4;
unsigned char displayPointer = 0; // for interrupt use...
unsigned char buffer1[4]; // buffer for screen, was 32 for matrix (4x8=32 and each character is built from 8x8 block, due 8x8 matrix) but for 7 segments, each character is 1x8 and screen is 4 digits so buffers are 4
unsigned char backbuffer[4]; // Spare screen for drawing on
unsigned char power[4] = {1, 2, 4, 8}; //B00000001,B00000010,B00000100,B00001000 for digits
// was: unsigned char power[8] = {128, 64, 32, 16, 8, 4, 2, 1};
ISR(TIMER1_COMPA_vect) // timer compare interrupt service routine, called every now and then, outside of loop
{
if (TIFR2) // Make sure its the timer interrupt.
{
setcolumn(displayPointer); //column scanning
setdata(buffer1[displayPointer]);
PORTD = (1 << PORTD3);
PORTD = (0 << PORTD3);
//digitalWrite(latchPin ,HIGH);
//digitalWrite(latchPin , LOW ); // STORECLOCK
if (++displayPointer == 4) //was 32, now 4 as buffer sizes changed too
{ displayPointer = 0;
} // 32 LED row sections in total
}
TIFR2 = 0; // Clear timer 2 interrupt flag
}
void setcolumn(unsigned char col) //loop that takes care of column scanning
{
signed char pos;
for (pos = 4; pos > -1; pos--) //was pos=32, but again, only 4 columns now so pos=4
{
if (col == pos)
{
PORTD = (1 << PORTD2); //digitalWrite(dataPin ,HIGH); //swapping these reversed unlit-lit digits
}
else
{
PORTD = (0 << PORTD2); //digitalWrite(dataPin ,LOW);
}
digitalWrite(clockPin , HIGH);
digitalWrite(clockPin , LOW);
}
}
void setdata(unsigned char dat) {
unsigned char pos;
for (pos = 0; pos < 8; pos++)
{
if (dat & 128) //these dat were 128, but changed them to 16 as 16=128/8
{
dat -= 128;
PORTD = (1 << PORTD2); //digitalWrite(dataPin ,HIGH); //swapping these caused whole screen to be reversed for lit-unlit segments
}
else
{
PORTD = (0 << PORTD2); //digitalWrite(dataPin ,LOW);} // PIN1 DATA pin
}
dat = dat * 2;
digitalWrite(clockPin , HIGH);
digitalWrite(clockPin , LOW);
}
}
void clr() //clear
{
int addr;
for (addr = 0; addr < 4; addr++) // Empty display buffer, reduced from 32 to 4
backbuffer[addr] = 0;
}
void Blit() //transfers data between display buffer to screen buffer
{
int addr = 0;
noInterrupts(); // disable all interrupts during setup
for (addr = 0; addr < 4; addr ++) //here also changed 32 to 4
{
buffer1[addr] = backbuffer[addr]; // put all data from display buffer to screen buffer
}
interrupts(); // enable all interrupts
}
void pixel(signed char x, signed char y, int cond)
{
unsigned char pix, msk;
if (x < 0 || y < 0) return; // outside drawing limits negative
if (x > 3 || y > 7) return; // outside drawing limits positive, changed x>31 to x>3
pix = power[y];
msk = backbuffer[x]; // get exsisting data
if (cond == 2)
pix ^= msk; // XOR data to screen
if (cond == 1)
{
pix = ~pix;
pix &= msk; // AND data to screen
}
if (cond == 0)
pix |= msk; // OR data to screen
backbuffer[x] = pix; // apply changes
}
void charput(unsigned char ch, signed char x, signed char y)
{
signed char y1;
unsigned char disp;
unsigned char disp2;
//for ( x1 = 0; x1 < 8; x1++) // eight rows, still eight bits to load so no changes
//{
// disp = font[x1 + (ch * 8)];
disp = font[ch];
Serial.println(disp,BIN); //display as BINARY format (Bxxxxxxxx) as fontmap is in binary format
for (y1 = 0; y1 < 8; y1++) // eight pixels
{
disp2 = disp & power[y1];
if (disp2 > 0)
{
//pixel(x + x1, y + y1, 0); // OR the pixel to the display buffer
pixel(x, y + y1, 0); // OR the pixel to the display buffer
}
}
//}
}
void strput(const char* ch, signed char x, signed char y)
{
int addr;
while (*ch )
{
charput(*ch++, x, y); // write a string to the display buffer
x += 7;
}
}
void setup() //setup runs once
{
Serial.begin(9600);
signed char cntr;
noInterrupts(); // disable all interrupts during setup
DDRD = DDRD | B11111100; //port registers used to set pin directions
TCCR1A = 0;
TCCR1B = 0;
TCNT1 = 0;
OCR1A = 260; // compare match register value, was 31 for matrix (1920hz= 60hzx*32) but for now there's only 4 columns so: 4*60hz=240hz; 16mhz/256/240=260
TCCR1B |= (1 << WGM12); // CTC mode, free-running, clear on match
TCCR1B |= (0 << CS10); // 256 prescaler
TCCR1B |= (0 << CS11); // 256 prescaler
TCCR1B |= (1 << CS12); // 256 prescaler
TIMSK1 |= (1 << OCIE1A); // enable timer compare interrupt
interrupts(); // enable all interrupts
} // End main
void loop() //main loop
{
clr();
charput(5, 0, 0); //test string to draw on segments //should show character 5 on hundreds digit
Blit();
}