Trung
New Member
1. Traffic light
I use the pic 16f84 to control three leds with three different color as the system traffic light. The diagram bellow help you see clearly:
Red light
Yellow light
Blue light
* Code for projects
I use the pic 16f84 to control three leds with three different color as the system traffic light. The diagram bellow help you see clearly:
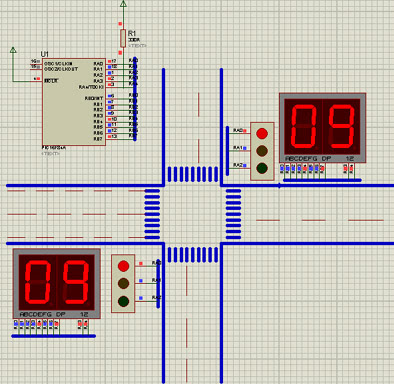
Red light
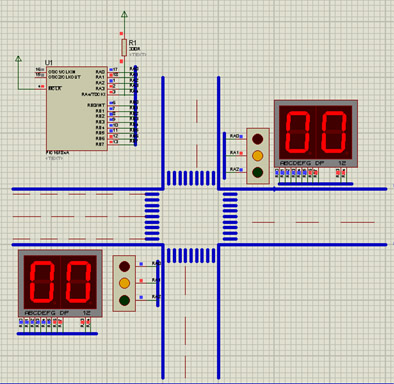
Yellow light
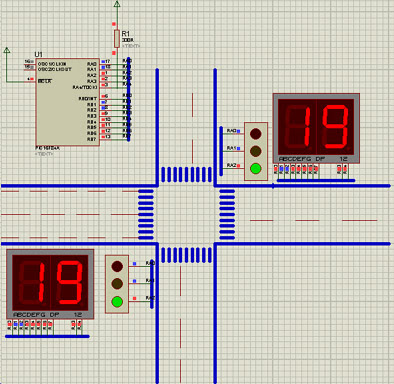
Blue light
* Code for projects
HTML:
/*Author: Huynh Minh Trung*/
unsigned int mask(unsigned short num) {
switch (num) {
case 0 : return 0xc0;
case 1 : return 0xf9;
case 2 : return 0xa4;
case 3 : return 0xb0;
case 4 : return 0x99;
case 5 : return 0x92;
case 6 : return 0x82;
case 7 : return 0xf8;
case 8 : return 0x80;
case 9 : return 0x90;
} //case end
}//~
int digit_no, digit10, digit1, digit, i;
void interrupt() {
if (digit_no==0) {
PORTA.F3 = 0; // Turn off all 7seg displays
PORTB = digit1; // send mask for ones digit to PORTD
PORTA.F3 = 1; // turn on 1st 7 seg., turn off 2nd
digit_no = 1;
} else {
PORTA.F4 = 0; // Turn off all 7seg displays
PORTB = digit10; // send mask for tens digit to PORTD
PORTA.F4 = 1; // turn on 2nd 7 seg., turn off 1st
digit_no = 0;
}
TMR0 = 0; // clear TMRO
INTCON = 0x20; // clear TMR0IF and set TMR0IE
}
void hienthi(){
digit = i % 10u;
digit1 = mask(digit); // prepare ones digit
digit = (char)(i / 10u) % 10u;
digit10 = mask(digit); // prepare tens digit
}
void main(){
OPTION_REG = 0x80; // Timer0 settings
TMR0 = 0;
INTCON = 0xA0; // Disable PEIE,INTE,RBIE,T0IE
TRISA=0X00;
PORTA=0;
TRISB=0X00;
PORTB=0;
do{
PORTA=0;
i=10;
for(i;i>=0;i--){
hienthi();
PORTA.F0=1;
delay_ms(1000);
}
PORTA=0;
i=3;
for(i;i>=0;i--){
hienthi();
PORTA.F1=1;
delay_ms(1000);
}
PORTA=0;
i=20;
for(i;i>=0;i--){
hienthi();
PORTA.F2=1;
delay_ms(1000);
}
}while(1);
}